Error handling in Python improves the robustness of the code, protecting it from possible errors that might cause the program to exit unexpectedly.
With the help of examples, you will learn error and exception handling in Python programs using try
, except
, and finally statements.
What is Error Handling In Python
When your program encounters an error (something in the program goes wrong), Python raises several built-in exceptions.
When one of these exceptions occurs, the Python parser terminates the current process and transfers control to the calling process before the exception is handled. The program will crash if it is not handled correctly.
Consider a program in which function A calls function B, which calls function C. If an exception occurs in function C but is not handled there, the exception is passed to B, who then passes it on to A.
If the error
is not handled, an error message appears, and our program comes to a halt.
How to Do Error Handling in Python
try
statement. The try
clause contains the critical operation that can cause an exception. The except clause includes the code that handles exceptions.
As a result, after we have caught the exception, we can select which operations to perform. Here’s an easy case.
Python
# import module sys to get the type of exception import sys randomList = ['a', 0, 2] for entry in randomList: try: print("The entry is", entry) r = 1/int(entry) break except: print("Oops!", sys.exc_info()[0], "occurred.") print("Next entry.") print() print("The reciprocal of", entry, "is", r) Result
Output
The entry is a Oops! <class 'ValueError'> occurred. Next entry. The entry is 0 Oops! <class 'ZeroDivisionError'> occured. Next entry. The entry is 2 The reciprocal of 2 is 0.5
We loop through the values of the randomList list in this program. As previously stated, the try block contains the code that may trigger an exception.
If no exceptions occur, the except block is bypassed and normal flow resumes (for last value). However, if an exception occurs, the except block will catch it (first and second values).
Using the exc info() function in the sys module, we print the name of the exception. We can see that 0 causes ZeroDivisionError and a causes ValueError.
Since any exception in Python is derived from the base Exception class, we can also accomplish the above task as follows:
Python
# import module sys to get the type of exception import sys randomList = ['a', 0, 2] for entry in randomList: try: print("The entry is", entry) r = 1/int(entry) break except Exception as e: print("Oops!", e.__class__, "occurred.") print("Next entry.") print() print("The reciprocal of", entry, "is", r) This program produces the same results as the previous one shown above.
Error Handling Python Try Except
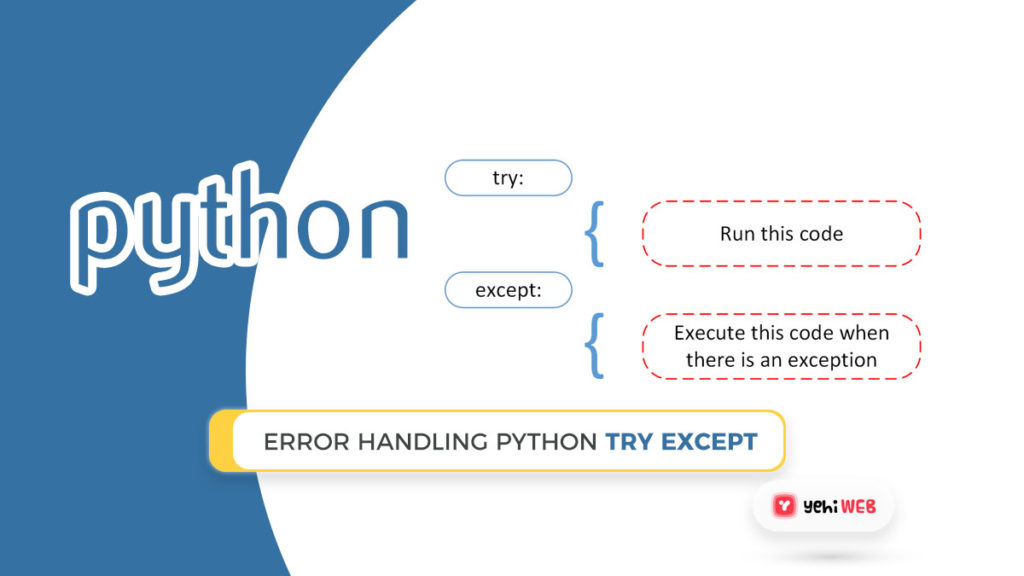
Exceptions are caught and handled in Python using the try
and except
block. Python treats the code after the try
statement as if it were a “normal” part of the program. The code after the except statement is the program’s response to any exceptions thrown by the try
clause before it.
Python throws an exception error when syntactically correct code encounters an error, as you saw earlier. If this exception error is not handled, the program will crash. Your program’s response to exceptions is determined by the except clause.
The try
and except
block can be better understood by using the following function:
Python
def linux_interaction(): assert ('linux' in sys.platform), "Function can only run on Linux systems." print('Doing something.')
You can only use the Linux interaction() function on a Linux system. If you call this function on a framework other than Linux, the assert will throw an AssertionError exception.
Python
try: linux_interaction() except: pass You handled the error in this case by issuing a pass. If you ran this code on a Windows system, you will get the following results: Shell You did not get anything. The program did not crash, which is a good thing. However, it would be interesting to see if some kind of exception existed while you run the code. To accomplish this, you can change the pass to something that will produce an informative message, such as: Python
Python
try: linux_interaction() except: print('Linux function was not executed') Run the following code on a Windows machine: Shell
Shell
Linux function was not executed
When an exception occurs in a program that uses this feature, the program will continue to run while still informing you that the function call failed.
The sort of error that was thrown as a result of the function call was not shown. You would need to catch the error that the feature throws in order to figure out what went wrong.
The following code illustrates how to capture the AssertionError and show it on the screen:
Python
try: linux_interaction() except AssertionError as error: print(error) print('The linux_interaction() function was not executed') On a Windows machine, this function returns the following: Shell
Shell
Function can only run on Linux systems. The linux_interaction() function was not executed
The AssertionError is the first message, and it informs you that You can only run the function on a Linux machine. The second message informs you of the function that was not executed.
You called a function that you wrote yourself in the previous example. You captured the AssertionError exception and printed it to the screen as you ran the function.
Another scenario in which you open a file and use a built-in exception is as follows:
Python
try: with open('file.log') as file: read_data = file.read() except: print('Could not open file.log') This block of code will output the following if file.log does not exist: Shell
Shell
Could not open file.log This is a helpful note, and our program will continue to run. There are a number of built-in exceptions that you can use here, according to the Python docs. The following is an example of an exception mentioned on that page:
FileNotFoundError
Raised when a file or directory is requested but doesn’t exist. Corresponds to errno ENOENT.
You may use the following code to capture this type of exception and print it to the screen:
Python
try: with open('file.log') as file: read_data = file.read() except FileNotFoundError as fnf_error: print(fnf_error) If file.log does not exist in this case, the output would be as follows: Shell
Shell
[Errno 2] No such file or directory: 'file.log' You can have multiple function called in your try clause to capture a variety of exceptions. It is worth noting that the code in the try clause will stop as soon as an exception is encountered. Warning: Catching Exception masks all errors...even the most unlikely ones. This is why bare except clauses in Python programs should be avoided. You can instead refer to specific exception types that you choose to capture and handle. In this guide, you can read more about why this is a good idea. Have a look at the code below. You first call the linux interaction() function before attempting to open a file: Python
Python
try: linux_interaction() with open('file.log') as file: read_data = file.read() except FileNotFoundError as fnf_error: print(fnf_error) except AssertionError as error: print(error) print('Linux linux_interaction() function was not executed') Running this code on a Windows machine would provide the following output if the file does not exist: Shell
Shell
Function can only run on Linux systems. Linux linux_interaction() function was not executed You immediately encountered an exception within the try clause and did not proceed to the part where you tried to open file.log. Now consider what happens when the code is executed on a Linux machine: Shell
Shell
[Errno 2] No such file or directory: 'file.log'
READ MORE
For 2021, the Best Password Managers
Key Takeaways:
- A try clause is executed before the first exception is encountered.
- You determine how the program responds to the exception in the except clause, also known as the exception handler.
- You may expect a variety of exceptions and tailor the program’s response to each one.
- Avoid bare except clauses.
Python: Catching Specific Exceptions
In the previous example, no specific exception was mentioned in the except clause.
This is not a good programming practice since it would capture all exceptions and handle each case the same. We can decide which exceptions to be caught by an except clause.
When an exception occurs, a try
clause may have any number of except
clauses to accommodate various exceptions, but only one can be executed.
In an except clause, we can assert several exceptions by using a tuple of values. The following is an example of a pseudo-code.
try:
# do something
pass
except ValueError:
# handle ValueError exception
pass
except (TypeError, ZeroDivisionError):
# handle multiple exceptions
# TypeError and ZeroDivisionError
pass
except:
# handle all other exceptions
pass
Python: Raising Exceptions
Exceptions are raised in Python programming when errors occur at runtime. We can also raise exceptions manually by using the raise keyword.
We can optionally pass values to the exception to get more information on why it raised it.
>>> raise KeyboardInterrupt
Traceback (most recent call last):
...
KeyboardInterrupt
>>> raise MemoryError("This is an argument")
Traceback (most recent call last):
...
MemoryError: This is an argument
>>> try:
... a = int(input("Enter a positive integer: "))
... if a <= 0:
... raise ValueError("That is not a positive number!")
... except ValueError as ve:
... print(ve)
...
Enter a positive integer: -2
That is not a positive number!
Python try/else clause
In certain cases, you might want to run a specific block of code if the code block inside the attempt completed successfully. You should use the optional else keyword with the try
statement in these situations.
Note: The preceding except clauses do not accommodate exceptions in the else clause.
# program to print the reciprocal of even numbers
try:
num = int(input("Enter a number: "))
assert num % 2 == 0
except:
print("Not an even number!")
else:
reciprocal = 1/num
print(reciprocal) Result In case we Pass an odd number:
Enter a number: 1 Not an even number! The reciprocal is computed and displayed if we pass an even number.
Enter a number: 4
0.25
If we pass 0 instead, we can get a ZeroDivisionError since the code block inside else is not handled by the except before it.
Enter a number: 0
Traceback (most recent call last):
File "", line 7, in
reciprocal = 1/num
ZeroDivisionError: division by zero
https://realpython.com/python-exceptions/#the-else-clause
Using Finally to Clean Up
Consider how you will have to implement some kind of cleanup action any time you ran the code. The final clause in Python allows you to do this.
Python
Python
try: linux_interaction() except AssertionError as error: print(error) else: try: with open('file.log') as file: read_data = file.read() except FileNotFoundError as fnf_error: print(fnf_error) finally: print('Cleaning up, irrespective of any exceptions.') All in the finally clause in the previous code will be executed. It makes no difference if you run across an exception in the try or else clauses. On a Windows machine, the following code will produce the following: Shell
Shell
Function can only run on Linux systems. Cleaning up, irrespective of any exceptions.
Final Thoughts
You learned how to raise, catch, and handle exceptions in Python after seeing the distinction between syntax errors and exceptions. You saw the following options in this article:
- You can throw an exception at any time with raise.
- You can use assert to check whether a condition is fulfilled and throw an exception if it isn’t.
- All statements are executed before an exception is encountered in the try clause.
- The exception(s) encountered in the try clause is captured and handled with except.
- else allows you to code parts that can only run if there are no exceptions in the try clause.
- Finally, with or without any previously found exceptions, you can run sections of code that should always run.
Hopefully, this article has given you a better understanding of the basic tools Python provides for dealing with errors and exceptions.